We are using recyclerview, for almost all of our android applications. It’s very useful to know about recyclerviews. in this post, I have explained all about animating recyclerview using the simple anim XML file.
Steps to animate recyclerview items in android
- Create animation XML files.
- Apply the animation to the layout container.
Create an animation XML file
To create an animation XML layout, Create an anim directory in your res folder.
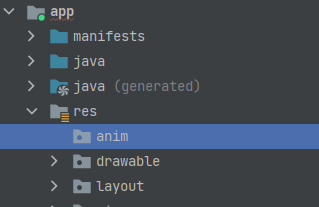
Then, create your animation XML file under the anim directory.
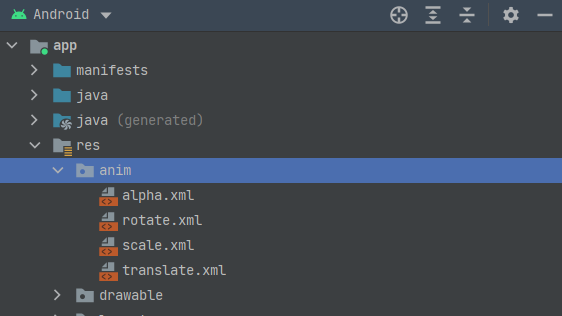
Apply the animation to the layout container
Once the animation XML file is created, we need to set the animation file into the layout root view. In our case, I have created adapter_movie.xml as an adapter layout for the recyclerview. And the root container is MatericalCardView and the id is container.
To add the animation to the layout we need to add,
holder.container.animation =
AnimationUtils.loadAnimation(holder.itemView.context, R.anim.scale)
We have a lot of animation available on android. below we will see some of the popular animations on android recyclerview.
Different animations on android
Alpha
An animation that controls the alpha level of an object. Useful for fading things in and out. This animation ends up changing the alpha property of a Transformation.
Parameters
fromAlpha: Float
– Starting alpha value for the animation, where 1.0 means fully opaque and 0.0 means fully transparent.
toAlpha: Float
– Ending alpha value for the animation.
alpha.xml
<?xml version="1.0" encoding="utf-8"?>
<alpha xmlns:android="http://schemas.android.com/apk/res/android"
android:fromAlpha="0.0"
android:toAlpha="1.0"
android:duration="500"
>
</alpha>
Output
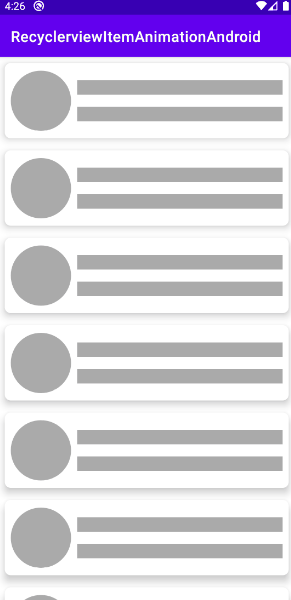
Rotate
An animation that controls the rotation of an object. This rotation takes place in the X-Y plane. You can specify the point to use for the center of the rotation, where (0,0) is the top-left point. If not specified, (0,0) is the default rotation point.
Parameters
fromDegrees: Float
– Rotation offset to apply at the start of the animation.
toDegrees: Float
– Rotation offset to apply at the end of the animation.
rotate.xml
<?xml version="1.0" encoding="utf-8"?>
<rotate xmlns:android="http://schemas.android.com/apk/res/android"
android:fromDegrees="180"
android:toDegrees="0"
android:pivotX="100%"
android:pivotY="100%"
android:duration="250">
</rotate>
output
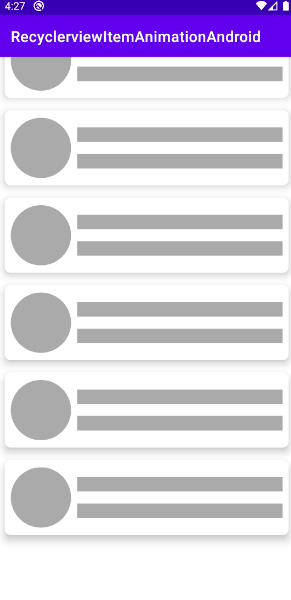
Scale
An animation that controls the scale of an object. You can specify the point to use for the center of scaling. Scale Animation is basically increasing or decreasing the size of the View.
Parameters
`fromX` | `float`: Horizontal scaling factor to apply at the start of the animation |
`toX` | `float`: Horizontal scaling factor to apply at the end of the animation |
`fromY` | `float`: Vertical scaling factor to apply at the start of the animation |
`toY` | `float`: Vertical scaling factor to apply at the end of the animation |
`pivotX` | `float`: The X coordinate of the point about which the object is being scaled, specified as an absolute number where 0 is the left edge. (This point remains fixed while the object changes size.) |
`pivotY` | `float`: The Y coordinate of the point about which the object is being scaled, is specified as an absolute number where 0 is the top edge. (This point remains fixed while the object changes size.) |
scale animation parameters
scale.xml
<?xml version="1.0" encoding="utf-8"?>
<scale xmlns:android="http://schemas.android.com/apk/res/android"
android:duration="500"
android:fromXScale="0"
android:fromYScale="0"
android:toXScale="1"
android:toYScale="1"
android:pivotY="50%"
android:pivotX="50%">
</scale>
output
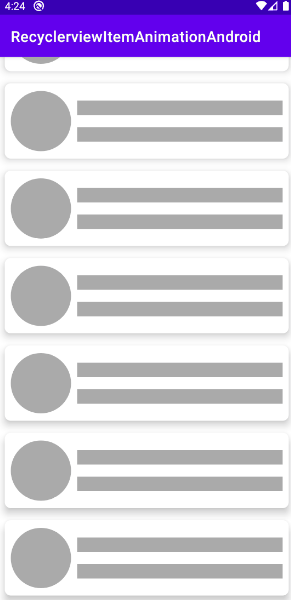
Translate
An animation that controls the position of an object.
Parameters
`fromXDelta` | `float`: Change in X coordinate to apply at the start of the animation |
`toXDelta` | `float`: Change in X coordinate to apply at the end of the animation |
`fromYDelta` | `float`: Change in Y coordinate to apply at the start of the animation |
`toYDelta` | `float`: Change in Y coordinate to apply at the end of the animation |
translate.xml
<?xml version="1.0" encoding="utf-8"?>
<translate
android:fromYDelta="100%"
android:fromXDelta="100%"
android:toYDelta="0%"
android:toXDelta="0%"
android:duration="500"
xmlns:android="http://schemas.android.com/apk/res/android" />
Output
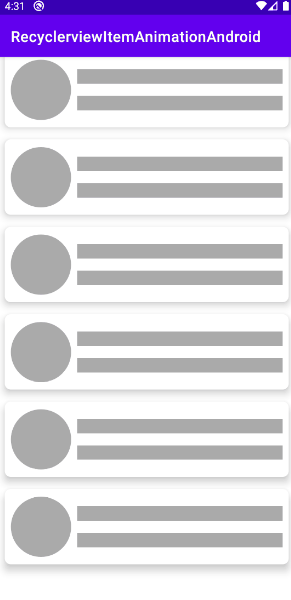
Interpolators
We have the last attribute which is interpolators. Interpolators are basically the behavior of the animations. Normally animations work on Linear Interpolator. That interpolator moves the view evenly over each frame of the animation.
- Accelerate Interpolator: This interpolator generates values that initially have a small difference between them and then ramps up the difference gradually until it reaches the endpoint. For example, the generated values between 1 -> 5 with accelerated interpolation could be 1 -> 1.2 -> 1.5 -> 1.9 -> 2.4 -> 3.0 -> 3.6 -> 4.3 -> 5. Notice how the difference between consecutive values grows consistently.
<?xml version="1.0" encoding="utf-8"?>
<set xmlns:android="http://schemas.android.com/apk/res/android"
android:interpolator="@android:anim/accelerate_interpolator"
android:duration="500">
<scale
android:fromXScale="0"
android:fromYScale="0"
android:toXScale="1"
android:toYScale="1"
android:pivotY="50%"
android:pivotX="50%"
>
</scale>
</set>
- Decelerate Interpolator: In the sense that the accelerate interpolator generates values in an accelerating fashion, a decelerate interpolator generates values that are “slowing down” as you move forward in the list of generated values. So, the values generated initially have a greater difference between them and the difference gradually reduces until the endpoint is reached. Therefore, the generated values between 1 -> 5 could look like 1 -> 1.8 -> 2.5 -> 3.1 -> 3.6 -> 4.0 -> 4.3 -> 4.5 -> 4.6 -> 4.7 -> 4.8 -> 4.9 -> 5. Again, pay attention to the difference between consecutive values growing smaller.
<?xml version="1.0" encoding="utf-8"?>
<set xmlns:android="http://schemas.android.com/apk/res/android"
android:interpolator="@android:anim/decelerate_interpolator"
android:duration="500">
<scale
android:fromXScale="0"
android:fromYScale="0"
android:toXScale="1"
android:toYScale="1"
android:pivotY="50%"
android:pivotX="50%"
>
</scale>
</set>
- Accelerate Decelerate Interpolator: This interpolator starts out with a slow rate of change and accelerates towards the middle. As it approaches the end, it starts decelerating, i.e. reducing the rate of change.
<?xml version="1.0" encoding="utf-8"?>
<set xmlns:android="http://schemas.android.com/apk/res/android"
android:interpolator="@android:anim/accelerate_decelerate_interpolator"
android:duration="500">
<scale
android:fromXScale="0"
android:fromYScale="0"
android:toXScale="1"
android:toYScale="1"
android:pivotY="50%"
android:pivotX="50%"
>
</scale>
</set>
- Anticipate Interpolator: This interpolation starts by first moving backward, then “flings” forward, and then proceeds gradually to the end. This gives it an effect similar to cartoons where the characters pull back before shooting off running. For example, generated values between 1 -> 3 could look like: 1 -> 0.5 -> 2 -> 2.5 -> 3. Notice how the first generated value is “behind” the starting value and how it jumps forward to a value ahead of the starting value. It then proceeds uniformly to the endpoint.
<?xml version="1.0" encoding="utf-8"?>
<set xmlns:android="http://schemas.android.com/apk/res/android"
android:interpolator="@android:anim/anticipate_interpolator"
android:duration="500">
<scale
android:fromXScale="0"
android:fromYScale="0"
android:toXScale="1"
android:toYScale="1"
android:pivotY="50%"
android:pivotX="50%"
>
</scale>
</set>
- Bounce Interpolator: To understand this interpolator, consider a meter scale that’s standing vertically on a solid surface. The starting value is at the top and the end value is at the bottom, touching the surface. Consider now, a ball that is dropped next to the meter scale. The ball on hitting the surface bounces up and down a few times until finally coming to rest on the surface. With the bounce interpolator, the generated values are similar to the list of values the ball passes by alongside the meter scale. For example, the generated values between 1 -> 5 could be 1 -> 2 -> 3 -> 4 -> 5 -> 4.2 -> 5 -> 4.5 -> 5. Notice how the generated values bounce.
<?xml version="1.0" encoding="utf-8"?>
<set xmlns:android="http://schemas.android.com/apk/res/android"
android:interpolator="@android:anim/bounce_interpolator"
android:duration="500">
<scale
android:fromXScale="0"
android:fromYScale="0"
android:toXScale="1"
android:toYScale="1"
android:pivotY="50%"
android:pivotX="50%"
>
</scale>
</set>
- Overshoot Interpolator: This interpolator generates values uniformly from start to end. However, after hitting the end, it overshoots or goes beyond the last value by a small amount and then comes back to the endpoint. For example, the generated values between 1 -> 5 could look like: 1 -> 2 -> 3 -> 4 -> 5 -> 5.5 -> 5.
<?xml version="1.0" encoding="utf-8"?>
<set xmlns:android="http://schemas.android.com/apk/res/android"
android:interpolator="@android:anim/overshoot_interpolator"
android:duration="500">
<scale
android:fromXScale="0"
android:fromYScale="0"
android:toXScale="1"
android:toYScale="1"
android:pivotY="50%"
android:pivotX="50%"
>
</scale>
</set>
- Anticipate Overshoot Interpolator: This interpolator is a combination of the anticipate and overshoot interpolators. That is, it first goes backward from the starting value, flings forward and uniformly moves to the endpoint, overshoots it, and then returns to the endpoint.
<?xml version="1.0" encoding="utf-8"?>
<set xmlns:android="http://schemas.android.com/apk/res/android"
android:interpolator="@android:anim/anticipate_overshoot_interpolator"
android:duration="500">
<scale
android:fromXScale="0"
android:fromYScale="0"
android:toXScale="1"
android:toYScale="1"
android:pivotY="50%"
android:pivotX="50%"
>
</scale>
</set>
That’s it. thank you for the reading. You can download this example on GitHub.
Leave a Reply