Contents
What is Kotlin?
Why kotlin in Android?
Kotlin internals
Java vs Kotlin
Features available in Kotlin
What is Kotlin?
Kotlin is a general purpose, open source, statically typed programming language for the JVM, Browser, Native, and Android that combines object-oriented and functional programming features.
In July 2011, JetBrains unveiled Project Kotlin, a new language for the JVM, which had been under development for a year.
One of the stated goals of Kotlin is to compile as quickly as Java. In February 2012, JetBrains open-sourced the project under the Apache 2 license.
Why kotlin on Android?
The name comes from Kotlin Island, near St. Petersburg. Andrey Breslav mentioned that the team decided to name it after an island just like Java was named after the Indonesian island of Java.
Kotlin is officially supported by Google for mobile development on Android. Since, the release of Android Studio 3.0 in October 2017, Kotlin is included as an alternative to the standard Java compiler. The Android Kotlin compiler lets the user choose between targeting Java 6 or Java 8 compatible bytecode.
Since, 7 May 2019, Kotlin is Google’s preferred language for Android app development.
Compatibility: It’s compatible with JDK 6, so older devices aren’t left behind.
Performance: It’s on par with Java.
Interoperability: It’s 100% interoperable with Java including annotations.
Compilation Time: There’s a little overhead on clean builds but it’s way faster with incremental builds.
Learning Curve: It’s easy to learn, especially for people used to modern languages. The Java to Kotlin converter in IntelliJ and Android Studio makes it even easier. You can also use a mix of Kotlin and Java in a project, so take your time learning Kotlin and add it in when you feel comfortable.
Kotlin Internals
Just like Java, Kotlin is a compiled language. This means before you can run Kotlin code, you need to compile it. Let’s discuss how the compilation process works and then look at the different tools that take care of it for you.
Kotlin source code is normally stored in files with the extension .kt. The Kotlin compiler analyzes the source code and generates .class files, just like the Java compiler does. The generated .class files are then packaged and executed using the standard procedure for the type of application you’re working on.
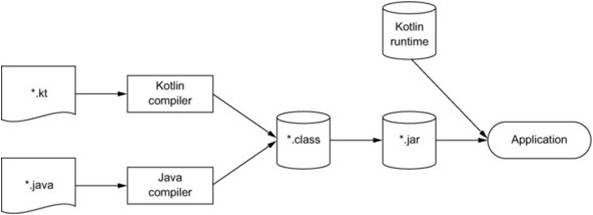
Java vs Kotlin
Print to Console
Java
System.out.print("Amit Shekhar");
System.out.println("Amit Shekhar");
Kotlin
print("Amit Shekhar")
println("Amit Shekhar")
Constants and Variables
var
(Mutable variable)
val
(Immutable variable)
Java
String name = "Amit Shekhar";
final String name = "Amit Shekhar";
Kotlin
var name = "Amit Shekhar"
val name = "Amit Shekhar"
Data Types
- The data type (basic type) refers to the type and size of data associated with variables and functions. The data type is used for the declaration of the memory location of the variable which determines the features of data.
- In Kotlin, everything is an object, which means we can call member functions and properties on any variable.
- Number
- Character
- Boolean
- Array
- String
Assigning the null value
Java
String otherName;
otherName = null;
Kotlin
var otherName : String?
otherName = null
Verify if the value is null
Java
if (text != null) {
int length = text.length();
}
Kotlin
text?.let {
val length = text.length
}
// or simply
val length = text?.length
Concatenation of strings
Java
String firstName = "Amit";
String lastName = "Shekhar";
String message = "My name is: " + firstName + " " + lastName;
Kotlin
var firstName = "Amit"
var lastName = "Shekhar"
var message = "My name is: $firstName $lastName"
New line in the string
Java
String text = "First Line\n" +
"Second Line\n" +
"Third Line";
Kotlin
val text = ""
|First Line
|Second Line
|Third Line
"".trimMargin()
Ternary Operations
Java
String text = x > 5 ? "x > 5" : "x <= 5";
String message = null;
log(message != null ? Message : "");
Kotlin
val text = if (x > 5) "x > 5" else "x <= 5"
val message: String? = null
log(message ?: "")
Bitwise Operators
Java
final int andResult = a & b;
final int orResult = a | b;
final int xorResult = a ^ b;
final int rightShift = a >> 2;
final int leftShift = a << 2;
final int unsignedRightShift = a >>> 2;
Kotlin
val andResult = a and b
val orResult = a or b
val xorResult = a xor b
val rightShift = a shr 2
val leftShift = a shl 2
val unsignedRightShift = a ushr 2
Check the type and casting
Java
if (object instanceof Car) {
}
Car car = (Car) object;
Kotlin
if (object is Car) {
}
var car = object as Car
// if object is null
var car = object as? Car
// var car = object as Car?
Multiple Conditions (Switch case)
Java
int score = // some score;
String grade;
switch (score) {
case 10:
case 9:
grade = "Excellent";
break;
case 8:
case 7:
case 6:
grade = "Good";
break;
case 5:
case 4:
grade = "OK";
break;
case 3:
case 2:
case 1:
grade = "Fail";
break;
default:
grade = "Fail";
}
Kotlin
var score = // some score
var grade = when (score) {
9, 10 -> "Excellent"
in 6..8 -> "Good"
4, 5 -> "OK"
in 1..3 -> "Fail"
else -> "Fail"
}
For-loops
Java
for (int i = 1; i <= 10 ; i++) { }
for (int i = 1; i < 10 ; i++) { }
for (int i = 10; i >= 0 ; i--) { }
for (int i = 1; i <= 10 ; i+=2) { }
for (int i = 10; i >= 0 ; i-=2) { }
for (String item : collection) { }
for (Map.Entry<String, String> entry: map.entrySet()) { }
Kotlin
for (i in 1..10) { }
for (i in 1 until 10) { }
for (i in 10 downTo 0) { }
for (i in 1..10 step 2) { }
for (i in 10 downTo 0 step 2) { }
for (item in collection) { }
for ((key, value) in map) { }
Collections
Java
final List<Integer> listOfNumber = Arrays.asList(1, 2, 3, 4);
final Map<Integer, String> keyValue = new HashMap<Integer, String>();
map.put(1, "Amit");
map.put(2, "Ali");
map.put(3, "Mindorks");
// Java 9
final List<Integer> listOfNumber = List.of(1, 2, 3, 4);
final Map<Integer, String> keyValue = Map.of(1, "Amit",
2, "Ali",
3, "Mindorks");
Kotlin
val listOfNumber = listOf(1, 2, 3, 4)
val keyValue = mapOf(1 to "Amit",
2 to "Ali",
3 to "Mindorks")
Defining methods
Java
void doSomething() {
// logic here
}
Kotlin
fun doSomething() {
// logic here
}
Variable number of arguments
Java
void doSomething(int... numbers) {
// logic here
}
Kotlin
fun doSomething(vararg numbers: Int) {
// logic here
}
Defining methods with return
Java
int getScore() {
// logic here
return score;
}
Kotlin
fun getScore(): Int {
// logic here
return score
}
// as a single-expression function
fun getScore(): Int = score
// even simpler (type will be determined automatically)
fun getScore() = score // return-type is Int
Features available in Kotlin
lazy Initialization
by lazy may be very useful when implementing read-only properties that perform lazy initialization in Kotlin.
by lazy { … } performs its initializer where the defined property is first used, not its declaration.
class Demo { val myName: String by lazy { "John" } }
Late Initialization
Late initialization variables are the developer’s promise that they will initialize the variable before accessing it.
lateinit var book: Book
Data Class
We frequently create a class to do nothing but hold data. In such a class some standard functionality is often mechanically derivable from the data. In Kotlin, this is called a data class and is marked as data.
data class Developer(val name: String, val age: Int)
- When we mark a class as a data class, you don’t have to implement or create the following functions as we do in Java.
- hashCode()
- equals()
- toString()
- copy()
The compiler automatically creates these internally, so it also leads to clean code.
Sealed Classes
Sealed classes are used for representing restricted class hierarchies wherein the object or the value can have value only among one of the types, thus fixing your type hierarchies. Sealed classes are commonly used in cases, where you know what a given value is only among a given set of options.
sealed class Operation {
class Add(val value: Int) : Operation()
class Substract(val value: Int) : Operation()
class Multiply(val value: Int) : Operation()
class Divide(val value: Int) : Operation()
}
Extension Functions
The extension functions in Kotlin allow us to extend the functionality of a class by adding new functions. The class doesn’t have to belong to us (could it be a third-party library) and also without require us to inherit the class.
fun Int.triple(): Int {
return this * 3
}
Conclusion
I hope you learned the basics of kotlin, and how to create functions and variables. Thanks for reading. let me know your comments below.
What’s Next,
Kotlin High Order Functions and Lambdas Explained
Kotlin Coroutines for Android Development
Kotlin Scope Functions Explained [Example]
Leave a Reply