Are you want to get the current location on android in jetpack compose? In this tutorial, I have explained how to get the current location on android using jetpack compose.
let’s jump into the coding, first, need to add the permission for the locations in the android manifest.xml
file.
Runtime location permissions
<uses-permission android:name="android.permission.ACCESS_COARSE_LOCATION" />
<uses-permission android:name="android.permission.ACCESS_FINE_LOCATION" />
<uses-permission android:name="android.permission.ACCESS_BACKGROUND_LOCATION"/>
To learn more about runtime permission on jetpack compose check out my other tutorial on Runtime Permission On Jetpack Compose.
Best ways to handle runtime permission on jetpack compose – Howtodoandroid
location permissions are the runtime permissions, So we need to ask the permission on runtime using rememberLauncherForActivityResult
.
val launcherMultiplePermissions = rememberLauncherForActivityResult(
ActivityResultContracts.RequestMultiplePermissions()
) { permissionsMap ->
val areGranted = permissionsMap.values.reduce { acc, next -> acc && next }
if (areGranted) {
locationRequired = true
startLocationUpdates()
Toast.makeText(context, "Permission Granted", Toast.LENGTH_SHORT).show()
} else {
Toast.makeText(context, "Permission Denied", Toast.LENGTH_SHORT).show()
}
}
So, we need to check the runtime permission with the click of the location button. Use ContextCompat.checkSelfPermission() to check the permission on runtime. Once the permission is granted we can start listening for the location.
Button(onClick = {
if (permissions.all {
ContextCompat.checkSelfPermission(
context,
it
) == PackageManager.PERMISSION_GRANTED
}) {
// Get the location
startLocationUpdates()
} else {
launcherMultiplePermissions.launch(permissions)
}
}) {
Text(text = "Get current location")
}
Getting current location
To get the current location, use getFusedLocationProviderClient() from the location service.
fusedLocationClient = LocationServices.getFusedLocationProviderClient(this)
Also, create the callback for the location update. we need to attach the location callback with the request location update function.
locationCallback = object : LocationCallback() {
override fun onLocationResult(p0: LocationResult) {
for (lo in p0.locations) {
// Update UI with location data
currentLocation = LocationDetails(lo.latitude, lo.longitude)
}
}
}
Now, we have created a location instance and callback for the location updates. let’s create the location request using these instances. Also, we can provide the location update interval and priority.
Use fusedLocationClient.requestLocationUpdates()
with location callback and request parameters to get the location update.
@SuppressLint("MissingPermission")
private fun startLocationUpdates() {
locationCallback?.let {
val locationRequest = LocationRequest.create().apply {
interval = 10000
fastestInterval = 5000
priority = LocationRequest.PRIORITY_HIGH_ACCURACY
}
fusedLocationClient?.requestLocationUpdates(
locationRequest,
it,
Looper.getMainLooper()
)
}
}
Once, we called this function, it will provide the location updates on the callback listener. Also, we need to start the location update at the start of the application and stop the location listener on the pause of the application.
override fun onResume() {
super.onResume()
if (locationRequired) {
startLocationUpdates()
}
}
override fun onPause() {
super.onPause()
locationCallback?.let { fusedLocationClient?.removeLocationUpdates(it) }
}
below, are the updated screenshots of the application.
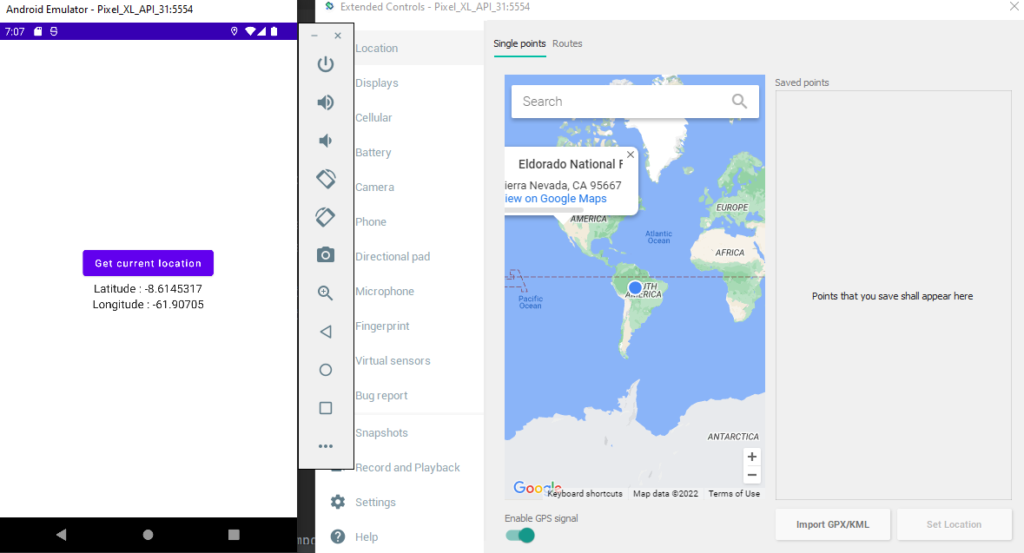
That’s it. thanks for reading. you can download this sample on GitHub.
Leave a Reply